For this project, I needed to write a method named polyspiral(Turtle t, int n, double base, int rounds)
that makes the turtle draw a spiral like the one shown below. The parameter n
specifies the shape of the spiral, the base
indicates the size of the smallest side of the spiral, and rounds
specifies the number of revolutions of the spiral.
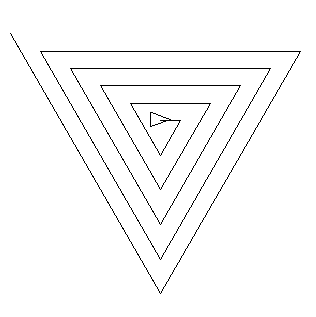
polyspiral (t, 3, 20, 5)
The Code
public class Polyspiral {
public static void main (String [] arguments) {
Turtle gabby = new Turtle (); // The turtle's name is gabby
polyspiral(gabby, n, 20, 5); // The triangle's parameters
}
Above is the main
method that houses all executions, including the call to the Turtle named gabby
. Starting at line 3, the Turtle gabby is created and then called and at line 4 the polyspiral
method, along with its parameters, is envoked. Notice a }
is missing for the class Polyspiral
, this is because the code will continue in the next code block.
public static void polyspiral (Turtle gabby, int n, double base, int rounds) {
for (int i = 0; i < (rounds * n); i ++) {
gabby.forward((i+1) * base); // The length of the polygon +1 after each increment
gabby.right(360.00/n); // The total angle (360.00) / total number of sides
}
// Now put the turtle back in it's original place
gabby.color("red"); // Changes the colour of the line
for (int j = (rounds * n); j > 0; j --) {
gabby.left(360.00/n);
gabby.backwards(j * base);
}
}
}
Above is the polyspiral()
method. Starting at line 1, the polyspiral
method is created and at line 2, we enter the first for-loop.
A for-loop
is a type of iteration (a loop) that takes a starting variable and value, and compares it to an ending condition. In each iteration, the value of the variable increases or decreases and is then compared again to the ending condition. If true, the loop continues. If false, the loop stops and we exit, moving on to the next line of code.
In this method, our starting variable and value is i = 1
and our ending condition is i < (rounds * n)
. Through each iteration, our value 1
is increased by i++
(which is just i = i +1
or +1
) and compared again to the condition. If true, gabby
moves forward and then to the right. If false, we exit the loop and move on to the next set of instructions.
Note that at lines 7 through 11, I use another for-loop
. This loop is used to put the turtle back in its original starting position. This is an extra step that is not particularly necessary, but it is in good practice to do so.